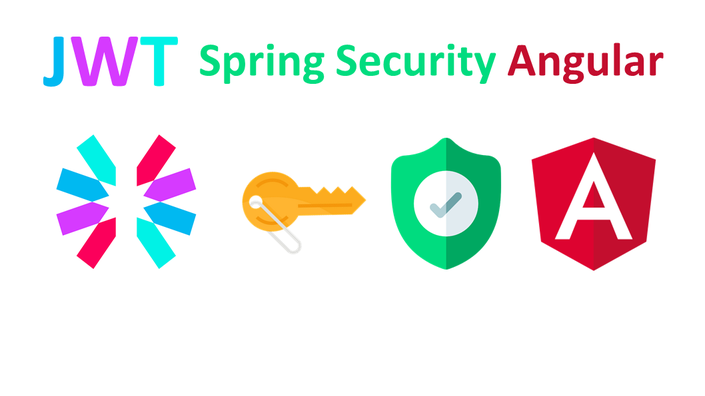
JSON Web Token (JWT) with Spring Security And Angular
Learn how to use JSON Web Token to secure REST applications, manage user roles and permissions, & way much more!
Watch Promo
According to jwt.io, "JSON Web Token (JWT) is an open standard (RFC 7519) that defines a compact and self-contained way for securely transmitting information between parties as a JSON object." In this course, you will understand and learn how to generate and sign a Json Web Token that users can use to securely access your RESTful application.
This course teaches how to use JWT (JSON Web Token) to embed users' roles and permissions to delegate user's authorization(what they can and can't do) in the application. This course also dives into defining and creating API, exposing API Endpoints over HTTP, and handling HTTP Requests and File Upload through API Endpoints. It covers testing API Endpoints (using an HTTP client - Postman and HTTPie).
200+ Lectures, 20+ Hours
Course Curriculum
-
PreviewWeb Application Security (2:29)
-
PreviewAuthentication and Authorization (2:33)
-
PreviewJson Web Toke (JWT) (2:56)
-
PreviewSecurity with Json Web Token (JWT) (3:19)
-
PreviewSecurity Constants (17:16)
-
PreviewJava JWT Library (4:35)
-
PreviewJWT Token Provider - Part 1 (30:56)
-
StartJWT Token Provider - Part 2 (12:17)
-
StartJWT Token Provider - Part 3 (11:59)
-
StartJWT Authorization Filter - Part 1 (10:22)
-
StartJWT Authorization Filter - Part 2 (10:53)
-
StartAuthentication Entry Point (11:11)
-
StartAccess Denied Handler (2:38)
-
StartUser Repository (4:01)
-
StartUser Details Service (17:27)
-
StartSecurity Configuration (15:47)
-
StartTesting Security Endpoint (11:20)
-
StartException Handling (4:56)
-
StartCustom Exception Classes (4:55)
-
StartCustom Exception Handling - Part 1 (5:16)
-
StartCustom Exception Handling - Part 2 (9:48)
-
StartCustom Exception Handling - Part 3 (7:56)
-
StartTesting Exception Handling (7:45)
-
StartOverride Spring Default White Label Error (23:38)
-
StartUser authorities (11:04)
-
StartUser Roles (6:07)
-
StartUser Registration - Part 1 (8:15)
-
StartUser Registration - Part 2 (9:34)
-
StartUser Registration - Part 3 (14:24)
-
StartUser Registration - Part 4 (5:36)
-
StartUser Registration - Part 5 (11:12)
-
StartCode Cleanup (9:04)
-
StartTesting User Registration (5:32)
-
StartDefining Brute Force Attack (2:37)
-
StartBrute Force Attack Cache - Part 1 (8:48)
-
StartBrute Force Attack Cache - Part 2 (11:05)
-
StartAuthentication Failure Listener (6:13)
-
StartAuthentication Success Listener (4:08)
-
StartValidating User Login (8:47)
-
StartTesting Brute Force Attack (10:27)
-
StartIntroduction (3:28)
-
StartTesting User Registration (9:37)
-
StartTesting User Login (8:54)
-
StartTesting User Add (9:10)
-
StartTesting User Update (5:10)
-
StartTesting User Find (3:00)
-
StartTesting User List (7:34)
-
StartTesting User Reset Password (5:49)
-
StartTesting User Delete (11:45)
-
StartTesting User Image Update (6:20)
-
StartCreating Service (5:19)
-
StartConfiguring HTTP and Environment Variable (5:39)
-
StartLogin HTTP Service Call (9:59)
-
StartCreating User Class (1:58)
-
StartRegistering User HTTP Call (2:20)
-
StartLogging User Out (3:43)
-
StartAdding Token to Cache (2:29)
-
StartAdding User to Cache (1:45)
-
StartRetrieving User from Cache (2:23)
-
StartLoading Token from Cache (2:46)
-
StartRetrieving Token from Cache (0:52)
-
StartChecking Logged in User (10:38)
-
StartListing User HTTP Call (3:25)
-
StartAdding User HTTP Call (3:30)
-
StartUpdating User HTTP Call (1:44)
-
StartResetting Password HTTP Call (3:11)
-
StartUpdating Profile Image HTTP Call (5:22)
-
StartDeleting User HTTP Call (2:13)
-
StartAdding Users to Cache (2:23)
-
StartRetrieving User from Cache (3:13)
-
StartAdding User Class Properties (2:53)
-
StartCreating Form Data (6:23)
-
StartCustom HTTP Response Mapping (8:05)
-
StartLogin Template - Part 1 (4:14)
-
StartLogin Template - Part 2 (2:19)
-
StartLogin Component - Part 1 (7:22)
-
StartLogin Component - Part 2 (6:07)
-
StartLogin Component - Part 3 (5:06)
-
StartLogin Component - Part 4 (4:25)
-
StartLogin Component - Part 5 (2:12)
-
StartAdding HTTP Header Type (2:15)
-
StartLogin Template - Part 3 (4:13)
-
StartLogin Template - Part 4 (5:39)
-
StartTesting Login - Part 1 (6:10)
-
StartAdding Notification Styles and Element (6:12)
-
StartTesting Login - Part 2 (4:49)
-
StartTesting Login - Part 3 (5:58)
-
StartTesting Login - Part 4 (6:17)
-
StartAdding New User Form (6:17)
-
StartJavaScript File Change Event (5:03)
-
StartGetting Profile Image and Save button (6:28)
-
StartSaving New User (7:50)
-
StartRefactoring and Testing Add New User (8:27)
-
StartAbout the Implementation (1:51)
-
StartSearch User - Part 1 (8:21)
-
StartSearch User - Part 2 (10:55)
-
StartOpen Edit Model Logic (7:20)
-
StartEdit User HTTP Call (4:34)
-
StartTesting Edit User (2:10)
-
StartDelete User HTTP Call (5:17)
-
StartTesting Delete User (4:13)
-
StartReset Password HTTP Call (6:30)
-
StartTesting Reset Password (8:50)
-
StartIntroduction (3:06)
-
StartMapping User Roles (2:44)
-
StartFetching Current User Role (4:34)
-
StartRole-based Security Management - Part 1 (6:36)
-
StartRole-based Security Management - Part 2 (6:23)
-
StartRole-based Security Management - Part 3 (6:51)
-
StartManaging Subscriptions (2:09)
-
StartManaging Subscriptions with Subsink (5:26)
-
StartIntroduction (4:56)
-
StartUsing AWS (4:07)
-
StartProvisioning EC2 Instance (7:10)
-
StartConfiguring EC2 Instance - Part 1 (6:37)
-
StartConfiguring EC2 Instance - Part 2 (7:38)
-
StartConfigure Spring Boot Application (7:30)
-
StartRunning in AWS (7:47)
-
StartDeploying Angular Application (13:51)
-
StartCreating Unix Service (16:56)
-
StartTesting in Production (4:08)
Course Prerequisites
Basic (web) development knowledge is required
Basic Spring Boot knowledge is required